Code minification is a fundamental technique for improving website performance. In this article we'll explain how code minification works, how it's different from text compression, and how you can set it up for your website.
What is code minification?
Minification is a way to reduce the download size of a web page. Less data to download means the page finishes loading faster and visitors spend less time waiting for content to appear.
How does minifying code work? It's a form of lossy compression, where unnecessary characters are removed from the code. The minified code will be harder for humans to read, but behaves the same as before while using less space. So from a behavior perspective the compression is lossless.
Content that is typically removed during minification includes:
- Code comments
- Whitespace (like line breaks or code indentation)
- Long variable names
Example of JavaScript minification
Let's take a look at what minification looks like in practice. Here's an example of what code typically looks like, with comments, clean formatting, and descriptive names for variables and functions.
/* Returns the sum of a and b */
function add(a, b) {
return a + b;
}
const sum = add(5, 6);
console.log(sum);
After minification the code looks like this:
function n(n,o){return n+o}const o=n(5,6);console.log(o);
We've gone from 113 characters to just 57!
Let's run that through an automatic code formatter like Prettier to restore the whitespace.
function n(n, o) {
return n + o;
}
const o = n(5, 6);
console.log(o);
Now we can see more clearly what has changed:
- The comment at the top has been removed, saving 32 bytes
- the
add
function has been renamed ton
, saving 4 bytes across both the definition and the references sum
has been renamed too
, saving 4 bytes
The remaining savings come from the removal of whitespace.
If you try this example yourself in a JavaScript minifier the function and variable might not be renamed. That's because in our simple example the add
and sum
definitions are declared in the top-level scope, which means other script might need acess to them.
Minifiers often provide a setting to control whether optimizations are applied to these definitions.
Example of CSS minification
Just like JavaScript, CSS code can also be minified.
.slider {
background: red;
color: white;
}
/* Another ruleset - more a thousand lines further down in the stylesheet */
.slider {
font-weight: bold;
color: blue;
}
After minification, the comments and whitespace are removed.
.slider{background:red;font-weight:700;color:#00f}
But the CSS minification also resulted in some other changes:
- the two rulesets with the same selector were combined into one
- the
color: white
rule was removed, since it was overridden bycolor: blue
bold
changed to700
, saving one character
Example of HTML minification
Finally, let's look at an example of minifying HTML.
<!-- Web Dev Languages -->
<ul
class="list
list--green"
>
<li>HTML</li>
<li>CSS</li>
<li>JavaScript</li>
</ul>
After minification, the comments and excess whitespace have been removed.
<ul class="list list--green"><li>HTML<li>CSS<li>JavaScript</ul>
What's the difference between code minification and text compression?
HTTP text compression with algorithms like GZIP and Brotli is another way to reduce the download size of a website resource without changing its behavior. How is text compression different from code minification?
While text compression works for every text document, code minifiers understand the meaning of the text they're compressing. They know that, in JavaScript, comments are wrapped in /* */
and removing them doesn't impact how the code runs. Minfiers also know that, in the language being minified, whitespace does not impact the behavior of the code.
That means that minification tools can apply different size reduction than generic text compression algorithms.
However, minification tools don't attempt to do the job of text compression. Instead, minification is additional layer in the process of reduce the size the code files on your website.
Developers (hopefully) write clear readable code. During a build step, minification tools analyze that code and return a smaller version with the same size. This minified code is uploaded to a server and, when the browser requests the file, the server compresses the code and sends it to the client. The browser can't run compressed code directly, so it needs to decompress it to obtain the minified version. The minified version can run in the browser without problems.
How does the size reduction differ between minification and compression?
How much do minification and compression reduce file size? And how much overlap is there between the two?
Let's measure this using Paul Calvano's Compression Level Estimator and the development React bundle.
Without text compression, minification reduces the file size from 88 kilobytes to just 26.5 kilobytes, a saving of 70%! Brotli compression by itself achieves a size reduction of 76%.
However, together the two methods have the biggest impact, achieving an overall size reduction of 90%.
What are the benefits minifying JavaScript and CSS code?
Code minification has a few benefits:
- Minified code can be downloaded faster
- Parsing minified code takes less CPU time
- Removing comments and variable names makes it harder for people to steal your code
Smaller code means visitors get to see content more quickly, plus you can improve metrics like the Largest Contentful Paint which impact SEO.
How to minify your code
If you're building a JavaScript application, you're probably using a build tool like Webpack or ESBuild. These tools typically provide built-in support for minification.
If you see that the output is not minified it might be because you've enabled a development build rather than a production build. For example, Webpack has a mode
option that determines whether code is minified. ESBuild has a minify
setting that enables minification when set to true
.
Many website platforms provide plugins for minification or minify code automatically. For example, you can install a Wordpress plugin to minify CSS and JavaScript code.
Can code minification be reversed?
Yes, many minifier tools can generate source maps which allow you to link each character in the minified code back to the original source code. That makes life easier for developers, as they don't have to investigate production bugs using just the minified source code.
However, source maps typically aren't published. If you still want to make the code slightly more readable you can use a code formatting tool like Prettier. While these tools can restore indentation they can't restore the original variable names.
Use Lighthouse audits to check if your code is minified
Google's Lighthouse tool automatically checks whether your website uses various performance techniques, including minification. There are two audits related to minifying code:
- Minify JavaScript
- Minify CSS
If you can't see these audits under Diagnostics you might be able to find them under Passed audits.
As you can see from these screenshots, Lighthouse includes a variety of "stackpacks" - special plugins that identify the platform your website is built on (the "stack") and give tailored advice. For example, for content management systems (CMSs) like Wordpress or Drupal you'll see specific recommendations on how to turn on minification.
Test and monitor the loading speed of your website
Minification is just one technique to make your website fast. Run a free website speed test to get more insight and recommendations for your website.
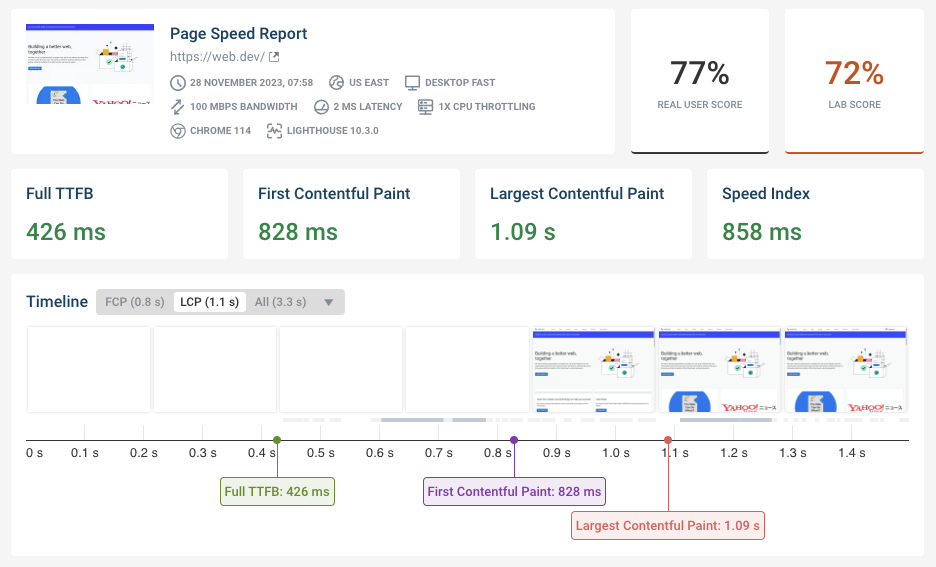
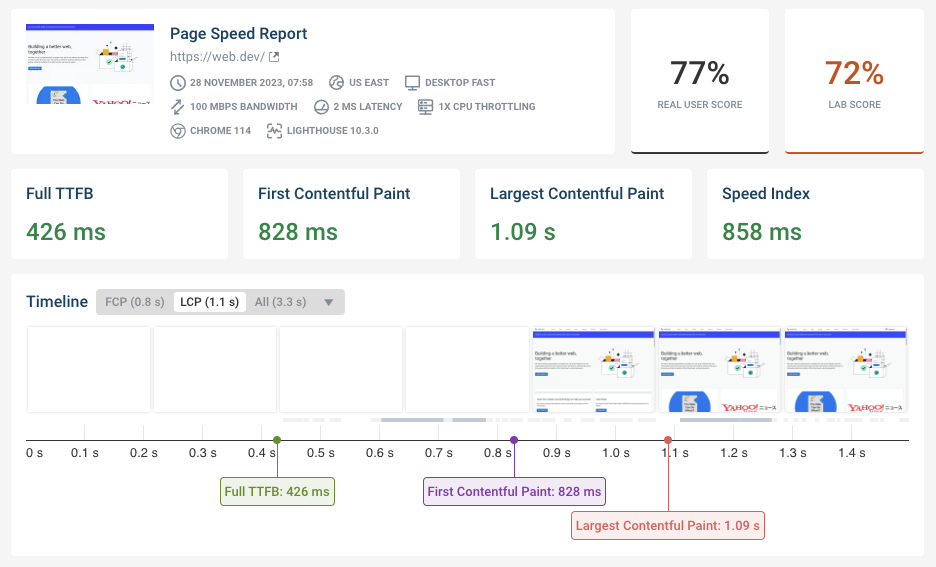
Run A Free Page Speed Test
Test Your Website:
- No Login Required
- Automated Recommendations
- Google SEO Assessment
If some of your code can be minified you'll find the "Minify CSS and JavaScript" recommendation at the bottom of the Overview tab in the test result.